$.ajax()方法概述
作用:发送Ajax请求
1 2 3 4 5 6 7 8 9 10 11
| $.ajax({ type: 'get', url: 'http://www.example.com', data: {name: 'zhangsan', age: '20'}, contentType: 'application/x-www-form-urlencoded', beforeSend: function(){ return false }, success: function(response){}, error: function(xhr){} })
|
实例,发送请求
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35
| var params = {name:"zhangsan", age:30} $('#btn').on('click',function(){ $.ajax({ type: 'get', url: 'http://localhost:8080/base', data: JSON.stringify(params), contentType: 'application/json', beforeSend: function(){ alert('请求即将发送') return false }, success: function(response){ console.log(response) }, error: function(xhr){ console.log(xhr) } }) })
|
serialize方法
作用:将表单中的数据自动拼接成字符串类型的参数
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31
| <form id="form"> <input type="text" name="username"> <input type="password" name="password"> <input type="submit" value="提交"> </form> <script> $("#form").on('submit',function(){ var params = serializeObject($(this)) console.log(params); return false; })
function serializeObject(obj){ var result = {}; var params = obj.serializeArray(); $.each(params, function(index, value){ result[value.name] = value.value }); return result; }
</script>
|
封装serializeObject函数
作用:输入的内容转化为json格式
1 2 3 4 5 6 7 8 9 10 11 12 13
| function serializeObject(obj){ var result = {}; var params = obj.serializeArray(); $.each(params, function(index, value){ result[value.name] = value.value }); return result; }
|
发送jsonp请求
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18
| <script> function fn(response){ console.log(response) } $('#btn').on('click',function(){ $.ajax({ url:'/jsonp', jsonp:'cp', jsonpCallback:'fn', dataType:'jsonp', success:function(response){ console.log(response) } }) }) </script>
|
$.get(),$.post()
1 2 3 4 5 6 7 8 9 10 11
| $('#btn').on('click',function(){ $.get('http://localhost:8080/abc',{username:'张三',password:'123456'},function(response){ console.log(response) }) $.get('http://localhost:8080/test',function(response){ console.log(response) }) $.post('http://localhost:8080/abc',{username:'zhangsan',password:'abcdef'},function(){ console.log(response) }) })
|
全局事件ajaxStart,ajaxComplete
也可以通过beforeSend实现,但是那样太麻烦
1 2 3 4 5 6 7 8
| $(document).on('ajaxStart', function(){ console.log('start') }) $(document).on('ajaxComplete',function(){ console.log('complete') })
|
进度条Nprocess
引入对应的文件
1 2
| <link rel="stylesheet" href="css/nprogress.css"> <script src="js/nprogress.js"></script>
|
编写代码:
1 2 3 4 5 6 7 8
| $(document).on('ajaxStart', function(){ NProgress.start() }) $(document).on('ajaxComplete',function(){ NProgress.done() })
|
RESTful API
一套关于设计请求的规范
GET:获取数据
POST:修改数据
PUT:更新数据
DELETE:删除数据
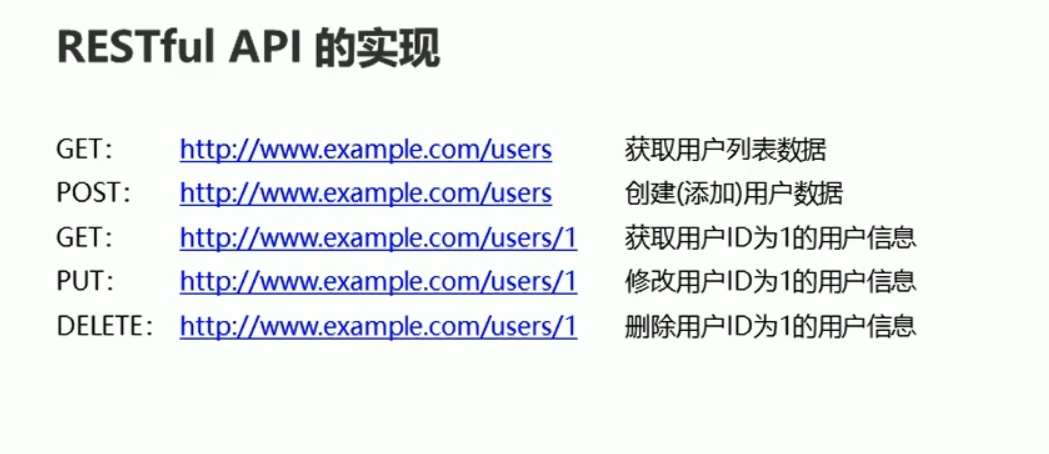
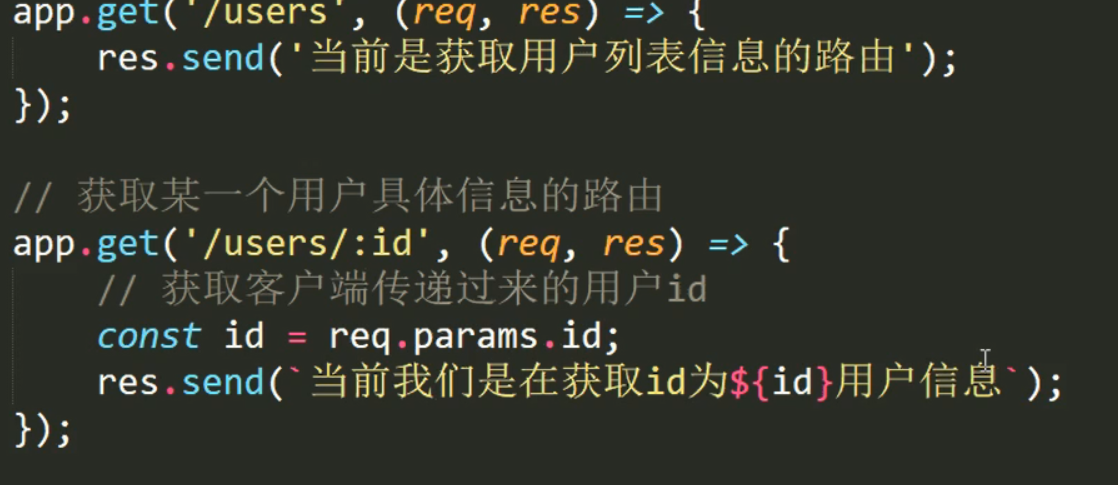
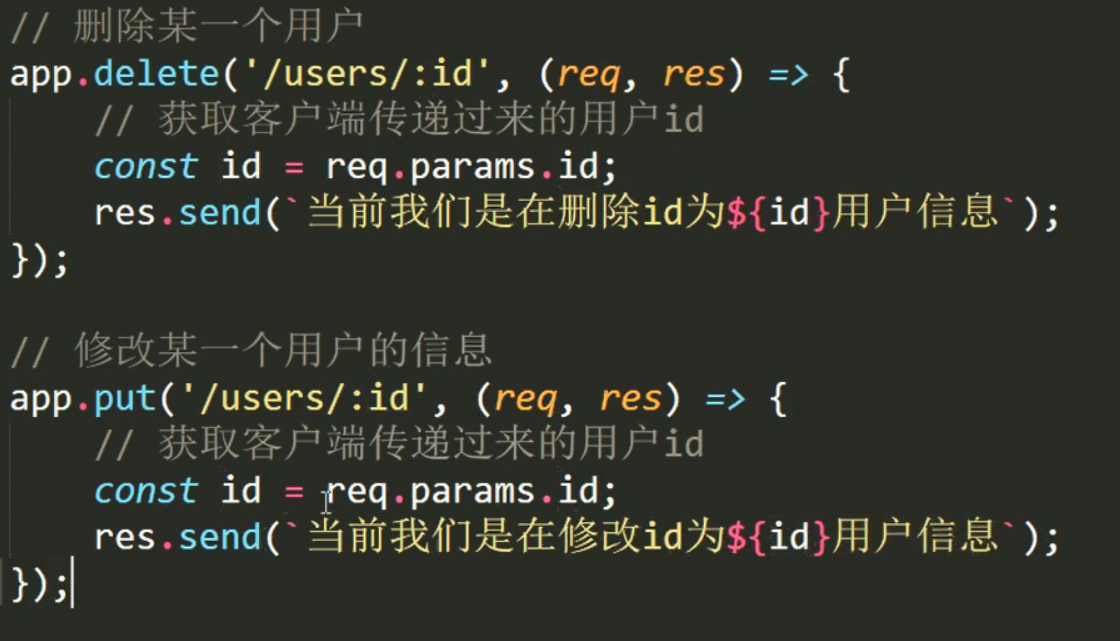